Given the root
of a Binary Tree, invert the tree, and return its root.
Example 1:
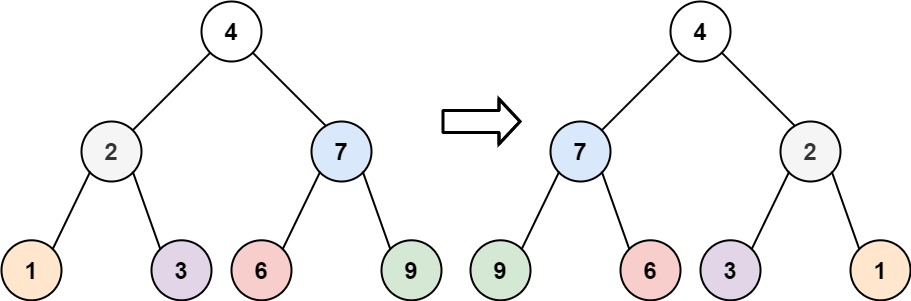
Input: root = [4,2,7,1,3,6,9] Output: [4,7,2,9,6,3,1]
Example 2:
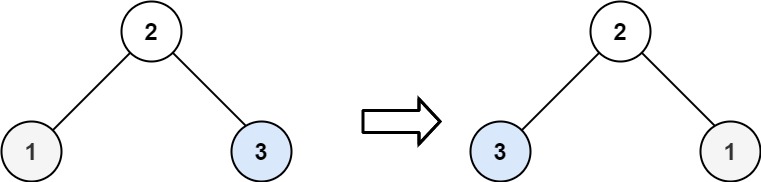
Input: root = [2,1,3] Output: [2,3,1]
Example 3:
Input: root = [] Output: []
NOTE:
- The number of nodes in the tree is in the range
[0, 100]
. -100 <= Node.val <= 100
Trivia:
This problem was inspired by this original tweet by Max Howell:Google: 90% of our engineers use the software you wrote (Homebrew), but you can’t invert a binary tree on a whiteboard so fuck off.
This problem is popular in LeetCode, StackOverFlow and several other forums - Ref 1 A collection of hundreds of interview questions and solutions are available in our blog at Interview Question Solutions
Solution:
No comments:
Post a Comment